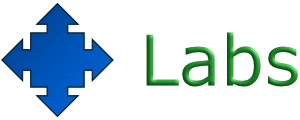

Mario Becroft <mb@tos.pl.net>
Sierpinski Triangle
An Example of Chaos Theory
Computer science and math are usually very exacting. However, there is one field of study known as Chaos Theory that is unlike traditional science. The idea behind chaos theory is that in large, complex systems that appear to be "chaotic," one can actually find order.
A classic Chaos Theory tale goes like this:
Somewhere in the Amazon, a butterfly flaps it wings causing a storm in New York that manages to delay an airplane landing at JFK causing a banker on board to miss an international monetary fund meeting causing a debate on the refinance of the Brazilian national debt to fail causing the brazilian government to go bankrupt causing an election resulting in a new president who bought victory with the promise of free land in the Amazon rainforest for the slum dwellers of Rio causing one lucky beneficiary name Jorge to claim, and then clear his land in the most efficient way available causing all the birds in their acre of the forest to migrate deeper into the heart of the jungle causing two thousand starving birds to be waiting as our original butterfly is just waking up to start the entire process all over again. This time the banker makes his meeting on time.
The "Sierpinski Triangle", introduced in 1916 by the Polish mathematician
Waclaw Sierpinski
(1882-1969), is a rather simple example of "order out of disorder." Suppose you
have an isosceles triangle and on one point
you write "1,2", on another point you write "3,4", and on the third
point you write "5,6". Pick a point somewhere inside the triangle.
Now roll a die. Now measure the distance from
your current position to the corner that has the number you rolled
on the die. Go half-way between your current position and the
corner and plot a point. Repeat the process indefinitely.
Eventually, you will have an image that looks like the triangle at the left.
Throughout the triangle, you can see areas that remain "untouched". If you
could take any corner of the triangle and enlarge it, you would see that it
would look look exactly like the whole triangle, with the same pattern of
"untouched" areas—so there is some order.
On the left, you can see the triangle get created using a simple JavaScript program.
If you want, you can also watch the Sierpinski Triangle render in a full screen view by clicking here.
Here is some some sample code that can produce the "Sierpinski Triangle."
#define MAX_TRIANGLE_X 320 // The size of the base
#define MAX_TRIANGLE_Y 200 // The height of the triangle
void SierpinskiTriangle()
{
int x, y; // Current position (x, y)
x = MAX_TRIANGLE_X / 2; // Select an initial position
y = 0;
while (1)
{
// Get a random number between 1 and 6
int die_roll = rand() % 6 + 1;
// Given the value of the die, select x and y
switch(die_roll)
{
case 1:
case 2:
x = (x + (MAX_TRIANGLE_X / 2)) / 2;
y = (y + 0) / 2;
break;
case 3:
case 4:
x = (x + 0) / 2;
y = (y + MAX_TRIANGLE_Y) / 2;
break;
case 5:
case 6:
x = (x + MAX_TRIANGLE_X) / 2;
y = (y + MAX_TRIANGLE_Y) / 2;
break;
}
// Draw the selected point
DrawPoint(x, y);
}
}